Private IPFS
IPFS has traditionally been a fully public network, so anything you pin can be accessed by anyone if they have the CID. While this is a benefit for a majority of blockchain applications, there are still cases where true privacy is needed. This is why Pinata has built Private IPFS, a new service that allows you to keep content private and only share when authorized.
Private vs Public Network
Everything in the SDK and API have been separated by two networks: public
and private
. This means files and groups will be in separate resources and will be accessed by designating the network in either the SDK method or API route.
Uploading
Listing and Querying
Groups
Accessing Private Files
With Public IPFS you can simply access a file through an IPFS Gateway with a CID. Since Private IPFS does not announce to the public IPFS network the only way you can access them is with a temporary access link. This can be generated with either the SDK or the API and set to expire after a designated number of seconds.
Example Apps
Pinata has built several example apps and tutorials you can reference to see how Private IPFS enables token gated experiences in blockchain and crpto contexts.
PinataCloud/concealmint-client
GitHub repo for CONCEALMINT, an app for creating and access Private NFTs
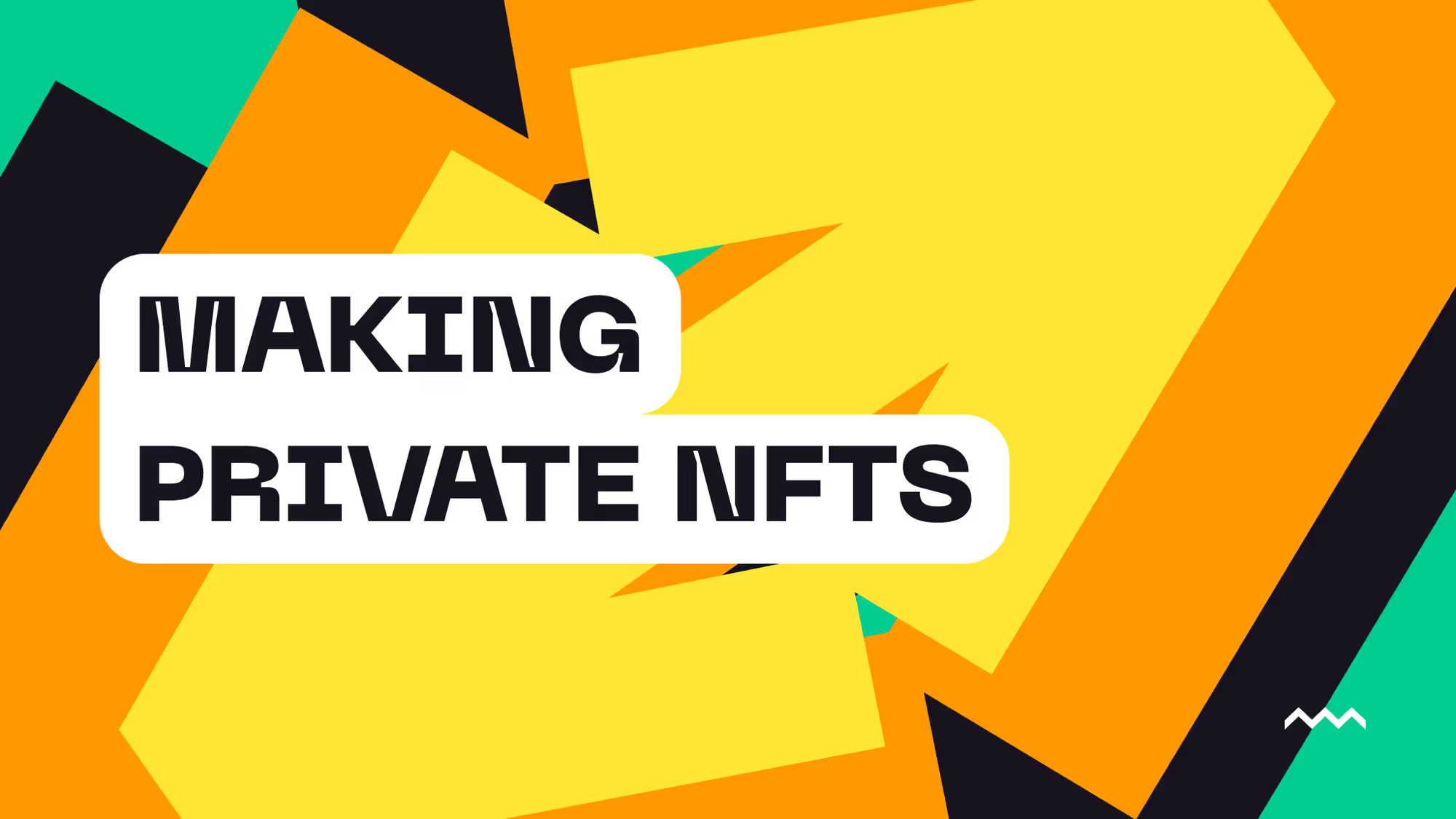
Making Private NFTs
By using Private IPFS you can gate acceess to files based on NFT ownership
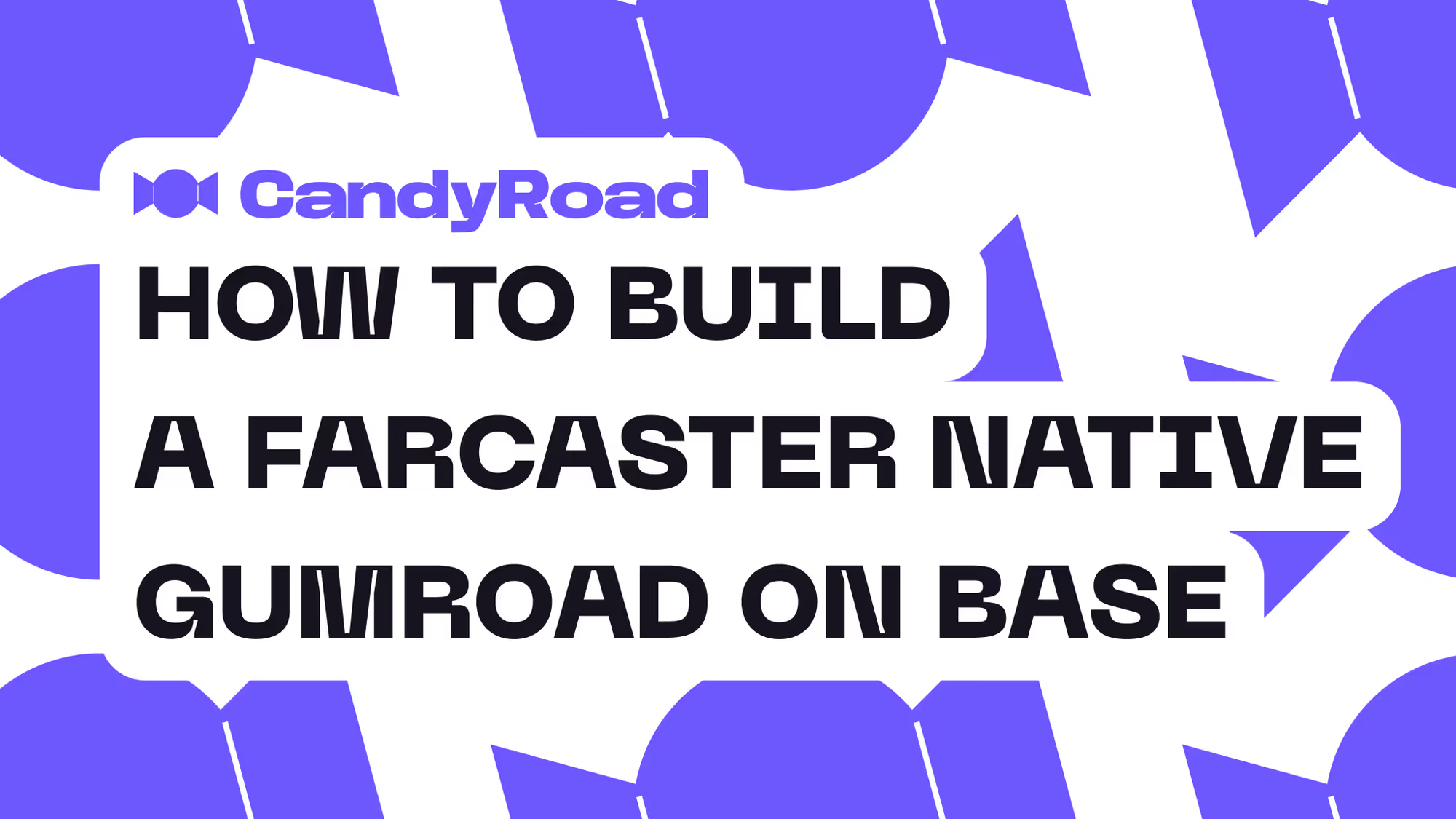
How to Build a Farcaster Native Gumroad on Base
Sell digital content inside Farcaster frames and keeping it secure through Private IPFS
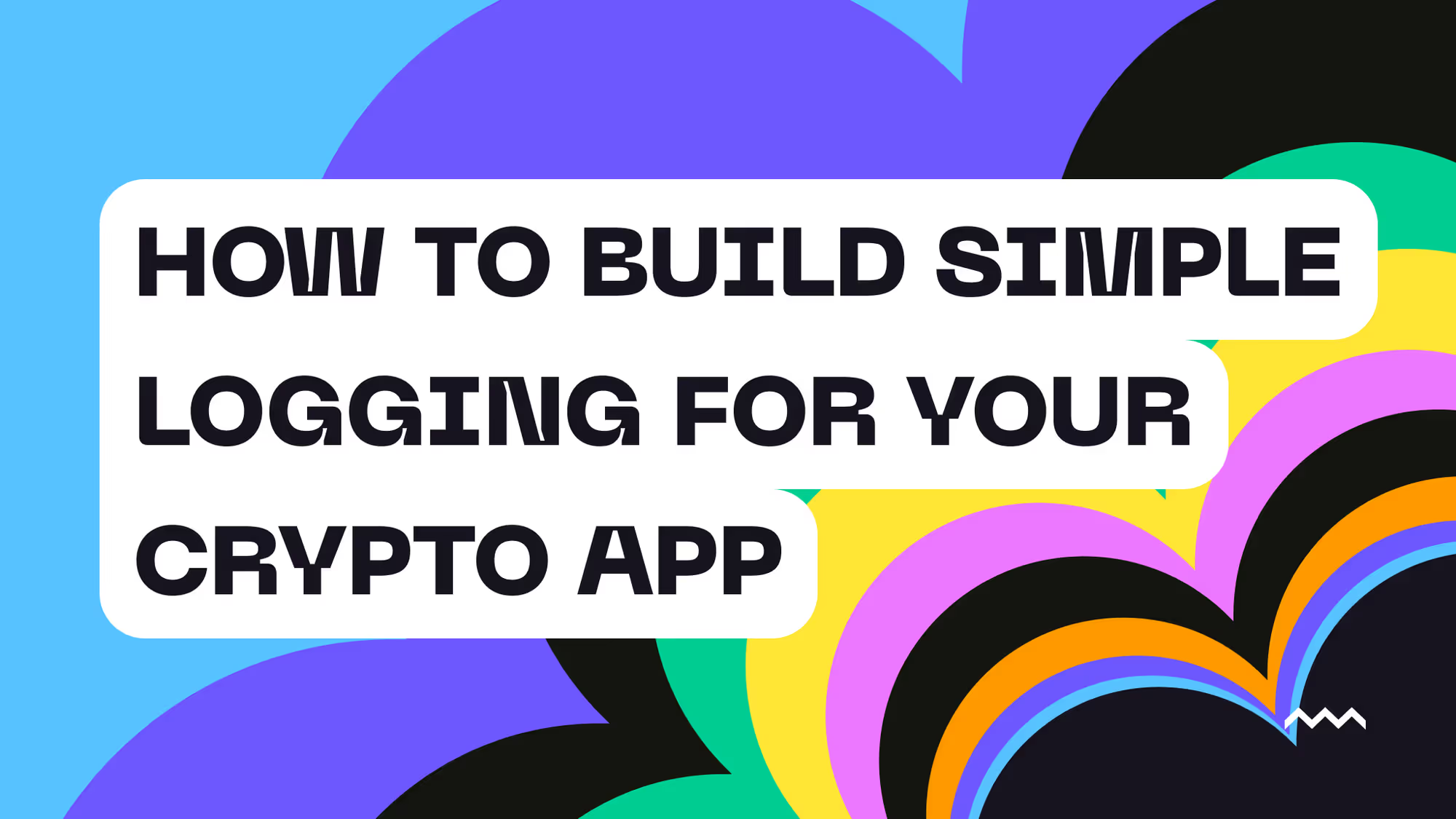
How To Build Simple Logging For Your Crypto App With Private IPFS
Add simple yet private logging to your crypto app using Private IPFS
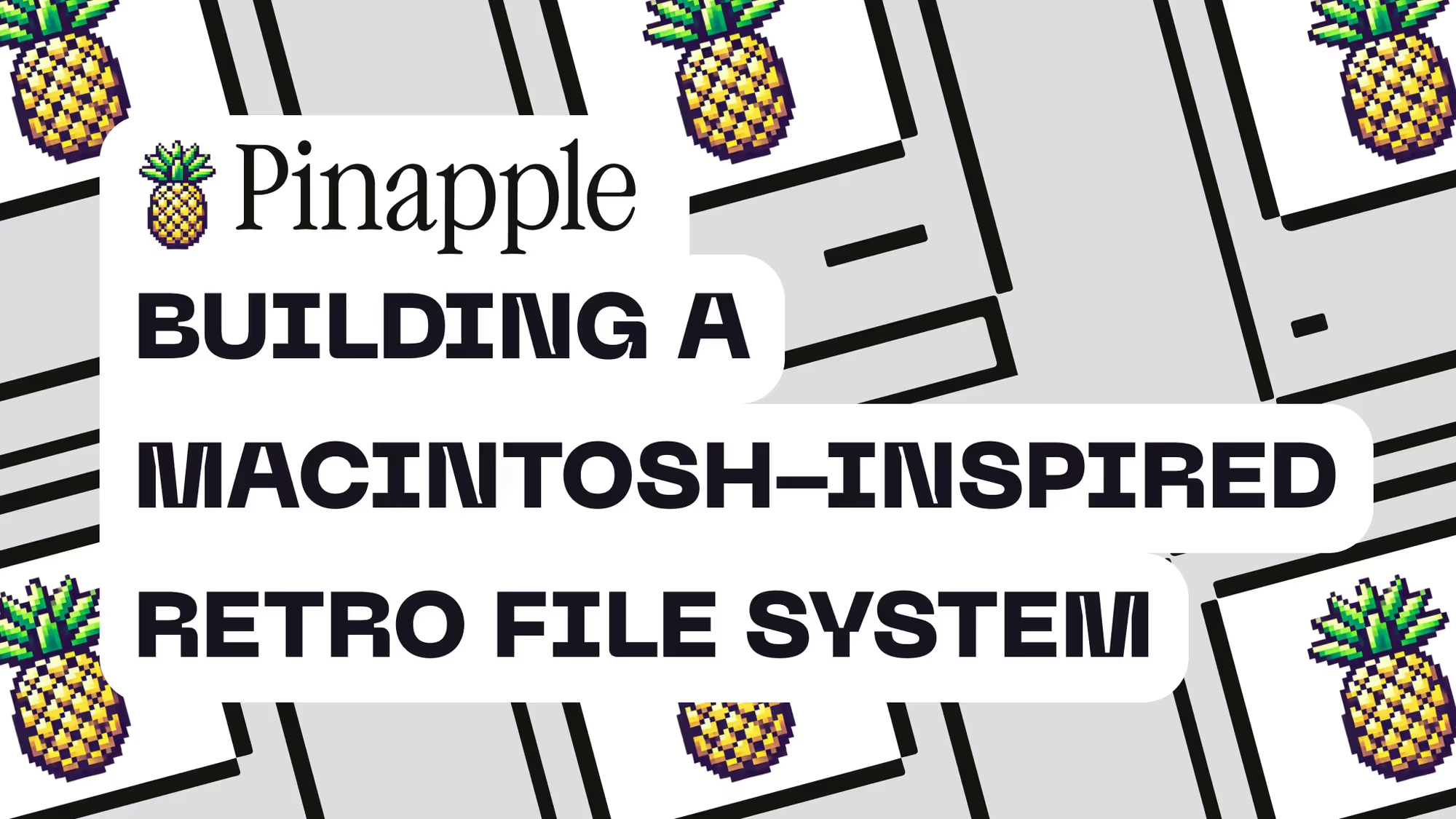
Building a Macintosh-Inspired Retro File System in the Browser
See the possibilities of file management using Groups and Private IPFS